1) 웹 크롤링(실시간 데이터 수집, Web Scraping)이란?
- 웹 사이트에서 원하는 정보를 추출하는 것을 의미한다.
- 보통 웹 사이트는 HTML 기반이므로 정보를 추출할 페이지에서 개발자모드 실행 후,
원하는 태그를 검색하는 스킬이 요구된다.
- 크롤링한 데이터를 소장, 활용하는 것은 불법이 아니지만 영리를 위한 목적 또는 배포시 문제가 될 수 있으니
이 경우에는 해당 사이트의 허락을 반드시 받아야한다.
2) 웹 크롤링 라이브러리
1. Jsoup
정적 데이터를 비교적 빠르게 수집할 수 있지만 브라우저가 아닌 HTTP Request를 사용하기 때문에
동적 데이터를 수집하기 위해서는 해당 서버의 인증키 요구 등 수집할 수 없는 경우가 많다.
2. Selenium
Jsoup에 비해 느리지만 브라우저 드라이버를 사용하여 동적 데이터도 수집이 가능하다.
3) 웹 크롤링 환경설치 방법 - Selenium 사용
1. 크롬 버전 확인하기
크롬 메뉴 -> 도움말 -> 크롬정보 -> 버전확인하기(앞에 있는 숫자 확인)
2. 버전에 맞는 크롬 드라이버 다운로드하기
https://chromedriver.chromium.org/downloads 접속하기 -> 드라이브 경로에다가 압축 해제하기
ChromeDriver - WebDriver for Chrome - Downloads
Current Releases If you are using Chrome version 92, please download ChromeDriver 92.0.4515.43 If you are using Chrome version 91, please download ChromeDriver 91.0.4472.101 If you are using Chrome version 90, please download ChromeDriver 90.0.4430.24 If y
chromedriver.chromium.org
3. 구글에 셀레니움 다운로드 검색 후 접속하기
SeleniumHQ Browser Automation
If you want to create robust, browser-based regression automation suites and tests, scale and distribute scripts across many environments, then you want to use Selenium WebDriver, a collection of language specific bindings to drive a browser - the way it i
www.selenium.dev
4. 상단에 다운로드 탭 클릭 후 원하는 언어의 다운로드 파일 다운로드 후 원하는 폴더 내에 압축 해제하기
https://www.selenium.dev/downloads/
Downloads
Firefox GeckoDriver is implemented and supported by Mozilla, refer to their documentation for supported versions. Internet Explorer Only version 11 is supported, and it requires additional configuration. Safari SafariDriver is supported directly by Apple,
www.selenium.dev
4) 웹 크롤링 (Selenium)사용예제 - 네이버에 접속하여 "마스크"단어 검색하여 상품명, 가격 가져오기
1. 이클립스 내에서 프로젝트 우클릭 후, Build Path 추가하기
다운 받은 jar 파일 모두 추가해주기
2. 이클립스 내 코드 입력해주기
package crawling;
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.chrome.ChromeOptions;
//네이버창 띄우기>검색창 태그 찾기>검색창 클릭>검색창에 마스크 문자열 입력>검색 버튼 태그 찾기>검색버튼 클릭
public class CrawlingTest {
public static String id = "webdriver.chrome.driver";
public static String path = "D:\\chromedriver.exe";
public static void main(String[] args) {
//크롬 드라이버 사용하기 위해 로딩
System.setProperty(id, path);
//크롬 브라우저를 열어줄 때 사용할 옵션들 설정할 수 있는 객체 만들기
ChromeOptions options = new ChromeOptions();
String url = "https://www.naver.com";
//아까 설정해준 옵션들을 기준으로 크롬 드라이버 실행하기
WebDriver driver = new ChromeDriver(options);
//실행된 드라이버로 주어진 url 접속시키기
driver.get(url);
//실행할 땐, 자바어플리케이션으로 실행하기
//url이 실행이 아래 코드보다 느리게 실행될 수도 있으니까 Thread 써주기
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
//페이지내에서.요소찾기("query"라는 아이디로); 라는 뜻
//driver.findElement(By.id("query"));
WebElement searchInput = driver.findElement(By.id("query"));
//검색창 클릭
searchInput.click();
//검색창에 마스크라는 문자열 전송하기
searchInput.sendKeys("마스크");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
//driver에서 "search_btn"라는 id를 가지고 있는 웹 요소 찾기
WebElement searchBtn = driver.findElement(By.id("search_btn"));
//검색 버튼 클릭
searchBtn.click();
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
//shop_guide_group라는 클래스를 가지고 있는 웹 요소 찾기
WebElement productDiv = driver.findElement(By.className("shop_guide_group"));
//네이버 쇼핑 div 안에서 ul 태그를 찾은 후, 그 안에 있는 box라는 클래스를 가지고 있는 웹 요소들 찾기(상품 li들)
List<WebElement> productList = productDiv.findElement(By.tagName("ul")).findElements(By.className("box"));
for(WebElement li : productList) {
//productList에서 li태그들 하나씩 가져오면서 반복 돌기
//상품 li에서 title 이라는 클래스를 가지고 있는 요소를 찾아서 그 안에 적힌 텍스트 긁어오기
String prodname = li.findElement(By.className("title")).getText();
//상품 li에서 price라는 클래스를 가지고 있는 요소 안의 strong 태그 요소 찾아서 그 안에 적힌 텍스트 긁어오기
String prodprice = li.findElement(By.className("price")).findElement(By.tagName("strong")).getText();
System.out.println("상품명 : "+prodname);
System.out.println("상품가격 : "+prodprice+"원");
System.out.println("===========");
}
}
}
3. 결과화면
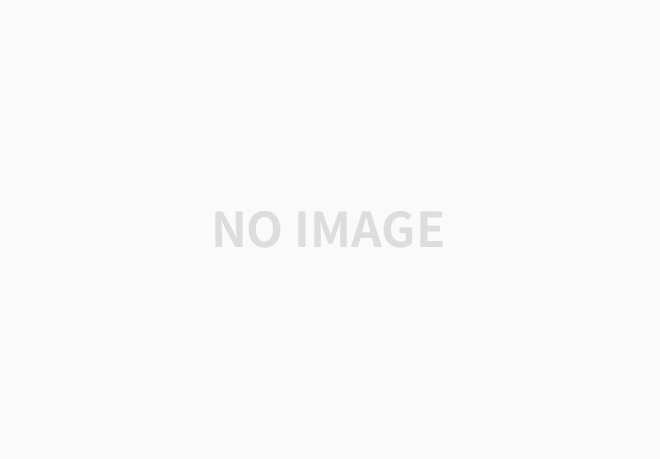
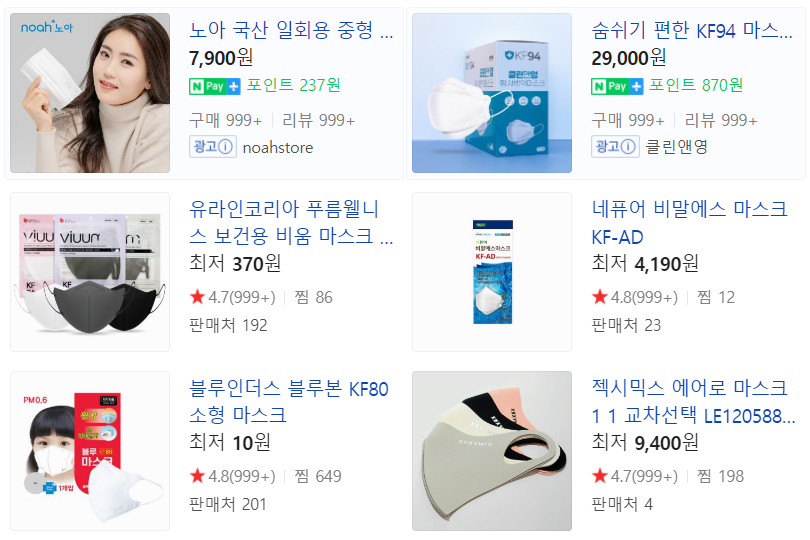
'Back-End > JAVA' 카테고리의 다른 글
[Java] MVC 모델 (0) | 2021.06.03 |
---|---|
[Java] 파일 입출력 (0) | 2021.06.02 |
[Java] Thread(쓰레드) (0) | 2021.06.01 |
[Java] 빠른 for문 (0) | 2021.06.01 |
[Java] HashMap (0) | 2021.06.01 |